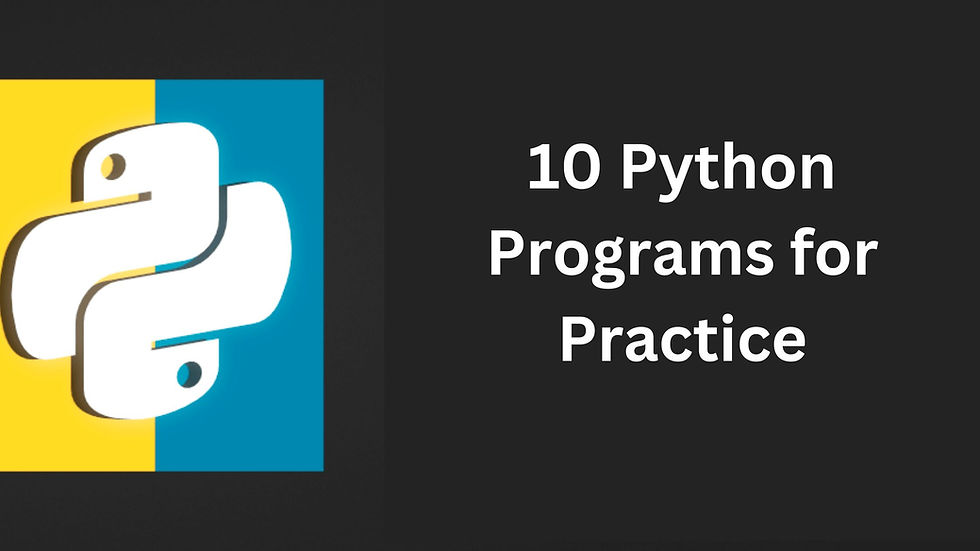
Python is a versatile and beginner-friendly programming language. It is widely used in web development, data science, machine learning, and other fields. Here are 10 Python programs for practice that will help you improve your skills and gain a deeper understanding of the language:
1. Print Statements and Variables:
Start with the basics by printing statements and variables to the console. This will help you get comfortable with the syntax and understand how to store and manipulate data in Python.
Python
print("Hello, World!")
message = "Welcome to Python programming!"
print(message)
2. Data Types and Operators:
Explore different data types in Python, such as integers, floats, strings, and booleans. Learn about arithmetic and comparison operators to perform calculations and comparisons.
Python
x = 10
y = 5
print(x + y)
print(x == y)
3. User Input and Conditional Statements:
Take user input using the input() function and use conditional statements like if, elif, and else to make decisions based on user input.
Python
name = input("Enter your name: ")
print("Hello, " + name + "!")
4. Lists and Loops:
Create and manipulate lists using indexing, slicing, and list methods. Learn about looping constructs like for and while to iterate through lists.
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
5. Tuples and Dictionaries:
Understand the difference between tuples and lists. Create and access elements in dictionaries, which are key-value pairs.
student = {"name": "Alice", "age": 15, "grade": "A"}
print(student["name"])
6. Functions and Modules:
Define and call functions to organize your code and reuse it. Import modules to access external libraries and functionalities.
def greet(name):
print("Hello, " + name + "!")
greet("Bob")
7. File Handling:
Read and write data from files using the open() function. Understand different file modes like r, w, and a.
with open("data.txt", "r") as file:
lines = file.readlines()
for line in lines:
print(line)
8. Regular Expressions:
Use regular expressions to search, match, and replace patterns in text. Learn about the re-module and its various functions.
import re
text = "The quick brown fox jumps over the lazy dog."
pattern = r"fox"
matches = re.findall(pattern, text)
print(matches)
9. Error Handling:
Handle errors using try and except blocks to catch exceptions and prevent crashes. Learn about different types of exceptions.
try:
x = int("abc")
except ValueError:
print("Invalid input")
10. Object-Oriented Programming:
Create classes and objects to model real-world entities and their interactions. Understand concepts like encapsulation, inheritance, and polymorphism.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person("John", 30)
print(person.name)
print(person.age)
These exercises will help you develop a solid foundation in Python and prepare you for more advanced programming tasks. Remember to practice regularly, explore different libraries, and seek help when needed.
Comments