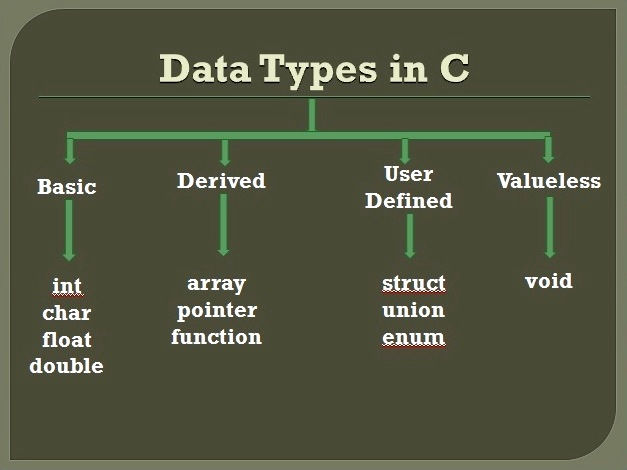
Data types are fundamental to any programming language, and in C, they play a crucial role in managing data efficiently and effectively. Data types determine the kind of values a variable can store, the amount of memory it occupies, and the operations that can be performed on it. In this article, we will delve into the world of data types in C, exploring their types, sizes, and usage.
Basic Data Types:
C provides several basic data types, categorized into the following groups:
Integer Types:
int: The most commonly used integer type, usually 2 or 4 bytes, depending on the platform.
short: A smaller integer type, typically 2 bytes.
long: A larger integer type, generally 4 bytes or more.
char: Represents a single character, typically 1 byte.
Floating-Point Types:
float: A single-precision floating-point type.
double: A double-precision floating-point type.
long double: An extended-precision floating-point type.
Void Type:
void: Represents the absence of type or value. It's often used as a return type for functions with no return value.
Modifiers:
C allows you to modify basic data types using various modifiers:
signed and unsigned: Used with integer types to specify whether they can hold both positive and negative values or only positive values.
short and long: Modify integer types to make them occupy less or more memory.
Size of Data Types:
The size of data types in C can vary depending on the compiler and platform. To determine the size of a data type on a particular system, you can use the sizeof operator. For example:
printf("Size of int: %lu bytes\n", sizeof(int));
User-Defined Data Types:
In addition to the basic data types, C allows you to define your own data types using structs, unions, and enums. These are essential for creating complex data structures and organizing data efficiently.
Structures (struct): Structures allow you to group variables of different data types together. For example, you can create a structure to represent a point in 3D space, containing x, y, and z coordinates.
Unions (union): Unions are similar to structures but occupy only as much memory as the largest member. They are often used when you need to represent a value that can be of different types at different times.
Enums (enum): Enums allow you to create a set of named integer constants, making your code more readable. For instance, you can define an enum for the days of the week.
Type Qualifiers:
C also provides type qualifiers that modify the behavior of data types:
const: Indicates that a variable's value cannot be changed after initialization.
volatile: Specifies that a variable's value can change at any time, without any action being taken by the code.
Defining and Using Data Types:
To define variables with different data types, you simply declare them using the data type's name. For example:
int age = 30;
double salary = 55000.50;
char grade = 'A';
You can also define your own data types using structures, unions, and enums. Here's an example of a structure:
struct Point {
int x;
int y;
};
And you can use this custom data type to declare variables:
struct Point p1, p2;
p1.x = 5;
p1.y = 10;
In summary, data types are essential in C for managing data efficiently and defining the nature of variables. Understanding the various basic data types, modifiers, user-defined data types, type qualifiers, and how to declare and use them is fundamental for any C programmer. This knowledge forms the basis for writing robust and efficient C code using a c compiler and is a critical step toward becoming a proficient C developer.
Comments